In this quick tip, we take a brief look at the popular Android image library, Picasso. It’s a simple and practical library created and maintained by Square. It is great for working with images in your Android projects.
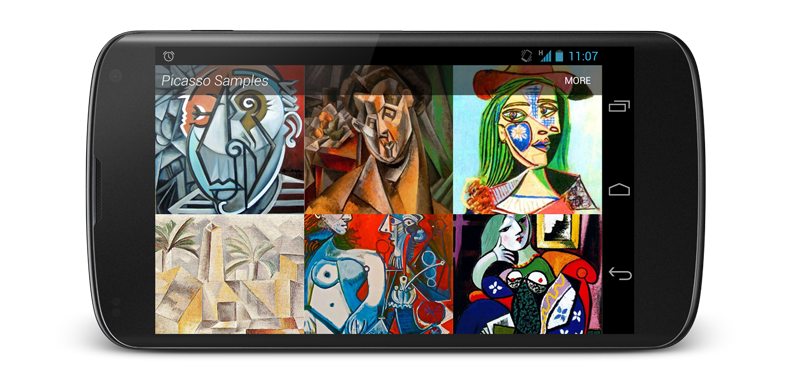
Working with Android image library Picasso – AndroidCodeGeeks.com
1. Introduction
Picasso is an image library for Android. It’s created and maintained by Square, and caters to image loading and processing. It simplifies the process of displaying images from external locations. In many cases only a few lines of code is required to implement this neat library.
Picasso shines for displaying remote images. The library handles every stage of the process, from the initial HTTP request to the caching of the image. This can be quite verbose when writing code to perform these actions yourself. In this quick tip, we look at a few common use cases.
2. Installation
Start by downloading the JAR file from Picasso’s website. Installing is done the usual manner. If you need help with this step, then take a look at this tutorial by Shane Condor and Lauren Darcey.
If you’re using Android Studio, then you can add
compile 'com.squareup.picasso:picasso:2.3.3'
to the build.gradle file in the dependency section.
3. Hands-On
Step 1: Create a new project
Create a new project in your IDE of choice. Make sure to select a blank Activity if you’re using Android Studio.
Step 2: Image Widget
Open the layout file for the main Activity. We need to add an ImageView
to the layout. It doesn’t need to be fancy. The following code snippet shows you what I mean.
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
Step 3: Add Picasso
Navigate to the main Activity file. Add the following code block to the onCreate method.
ImageView imageView = (ImageView) findViewById(R.id.imageView);
Picasso.with(this)
.load("https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg")
.into(imageView);
In the first line, we get a reference to the ImageView instance in the layout file. We then load an image into the image view using the Picasso library. We first specify the context by calling with and passing in the context. We then call the load method and supply it with the location of the image, a URL in this case. Finally, we tell Picasso where it should display the image when it’s fetched by calling into and pass in the imageView object.
Your IDE will ask you to import the Picasso library. However, to do this manually add the following import statement at the top of the Activity class.
import com.squareup.picasso.Picasso;
Step 4: Permissions
For Picasso to do its work, make sure to add <uses-permission android:name="android.permission.INTERNET" />
to your project’s manifest.
Step 5: Build and Run
That’s pretty much it. If you build and run the application, you should see the image load on the screen.
4. More Examples
Picasso has much more tricks up its sleeve. In the following example, we use Picasso to fetch a remote image and resize it before displaying it in an image view.
Picasso.with(this)
.load(https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg)
.resize(100, 100)
.into(imageView)
Picasso also supports transformations, such as rotation. In the next code snippet, we fetch a remote image and rotate it 180 degrees before displaying it in an image view.
Picasso.with(this)
.load("https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg")
.rotate(180)
.into(imageView);
If your application relies on remote assets, then it’s important to add a fallback in the form of a placeholder image. The placeholder image is shown immediately and replaced by the remote image when Picasso has finished fetching it.
Picasso.with(this)
.load(https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg)
.placeholder(R.drawable.image_name)
.into(imageView);
Picasso supports two types of placeholder images. We already saw how theplaceholder
method works, but there’s also an error
method that accepts a placeholder image. Picasso will try to download the remote image three times and display the error placeholder image if it was unable to fetch the remote asset.
Picasso.with(this)
.load(https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg)
.error(R.drawable.image_name)
.into(imageView);
Note that you can combine the placeholder
and error
methods as shown in the following code block.
Picasso.with(this)
.load(https://cms-assets.tutsplus.com/uploads/users/21/posts/19431/featured_image/CodeFeature.jpg)
.placeholder(R.drawable.image_name_default)
.error(R.drawable.image_name_error)
.into(imageView);
Conclusion
With Picasso being so simple to use, it’s definitely worth thirty minutes of your time. If you’re creating an app that frequently loads images, then Picasso could well make your life that little bit simpler.
Working with Android image library Picasso
1 comments:
Sign up for Free GPS tracking TrackMe | Global Positioning System | GPS Server
Post a Comment